Hello, World!
1 Python Basics
|
|
1.1 Introduction
A programming language is a computer system created to allow humans to precisely express algorithms to the computer using a higher level of abstraction. Python is one of the top ten most-used languages, and remains popular in developing web applications, in scientific computation, and as an introductory language for students. Organizations that use Python range from Google to NASA, DropBox to Industrial Light & Magic, and across the spectrum of casual, scientific, and artistic computer users.
Python emphasizes readability, and includes elements of the imperative, object-oriented, and functional programming paradigms, which will be explored later on. Python is an interpreted language, which for beginners means that Python instructions can be typed into an interactive prompt, or can be stored in a plain text file (called a “script”
) and run later. These instructions are evaluated and the commands are executed by the Python interpreter in something called the shell.
1.1.1 Hello, Python
A command, often called a statement, instructs the interpreter to do something. The first program described in many programming Language introductions is “Hello, World”. This simple program outputs a nominal greeting, demonstrating how a particular language produces a result, and also how a language represents text.
There are several aspects to note even in this simple Python statement. * First, print()
is a built-in function, a pre-defined operation that Python can use to produce output, a result of the program that will be made visible to the user. The print is followed by opening and closing parentheses; what comes between those parentheses is the value or arguments to be printed.
- Second, fixed values such as numbers, letters, and strings, are called constants or simple data type because their value does not change. String constants use single quotes
'
or double quotes"
in Python. The quotation marks in front of the capital'H'
and after the exclamation point denotes the beginning and end of a string of characters that will be treated as a value in Python.
Programming languages carry out their instructions very precisely. If a user makes subtle changes to the message between the starting and finishing quotation marks within the print statement, the resultant printed text will change accordingly. Notice that it is possible for statements to span more than one line using \
.
In Jupyter Notebook, the
1.1.1.1 Using string methods like a word processor
One of the simplest tasks you can do with strings is to change the case of the words in a string.
In this example, we have the lowercase string ‘hi python’. The method title()
appears after the string in the print()
call. A method is an action that Python can perform on a piece of data. The dot (.
) after the string tells Python to make the title()
method act on the string. Every method is followed by a set of parentheses that can accept arguments just like a function.
There are also other useful methods for string
print('hi python'.upper()) # change a string to all uppercase
print('Hello World'.lower()) # change a string to all lowercase
print(' hi python '.strip()) # remove extra whitespace on the right and left sides of a string
HI PYTHON
hello world
hi python
These example statements introduce another language feature. The #
symbol denotes the beginning of a comment, a human-readable notation to the Python code that will be ignored by the computer when executed. A high-level description at the top of a script introduces a human reader to the overall purpose and methodology used in the script. All of the characters to the right of the #
until the end of the line are ignored by Python.
1.1.2 Exercise 1: Complete the following items to make sure you correctly set up the environment.
- Open the explorer on the left-hand side
- Connect to the Python environment
- Create a new code cell below and write a code snippet that prints out “finish”. Execute the cell.
- Create a new script called “finish.py” and write a code snippet that prints out “finish”. Execute the script.
1.1.3 Operators and Expressions
Besides string, numbers are often used in programming. Python’s built-in operators allow numeric values to be manipulated and combined in a variety of familiar ways. Note that in Python, 2 + 3
is called an expression, which consists of values/operands (such as 2
or 3
) and operators (such as +
), and they are special statements! An expression is a combination of operators and operands that is interpreted to produce some other value.
1.1.3.1 Using operand like a calculator
# Integer
print(3+4) # Prints “7”, which is 3 plus 4.
print(5-6) # Prints “-1”, which is 5 minus 6
print(7*8) # Prints “56”, which is 7 times 8
print(45/4) # Prints “11.25”, which is 45 divided by 4, / is float(true) division
print(2**10) # Prints “1024”, which is 2 to the 10th power
7
-1
56
11.25
1024
Note that we used
**
to signify exponentiation, which can be somewhat surprising given that the caret symbol,^
, is often used for this purpose in some other programming languages. In Python, the caret operator belongs to XOR bitwise Boolean operations.
When an operation such as forty-five divided by four produces a non-integer result, such as 11.25
, Python implicitly switches to a floating-point representation. When purely integer answers are desired, a different set of operators can be used.
print(45//4) # Prints “11”, which is 45 integer divided by 4, // is floor(integer) division
print(45%4) # Prints “1”, because 4 * 11 + 1 = 45
11
1
The double slash signifies the integer floor division operator, while the percentage symbol signifies the modulus, or remainder operator. Taken together, we can read these calculations as, “Four goes into forty-five eleven times, with a remainder of one.”
String values also can be combined and manipulated in some intuitive ways.
helloworldhelloworldhelloworldhelloworld
The plus operator concatenates string values, while the multiplication operator replicates string values.
1.2 Variables
A variable is like a box in the computer’s memory where you can store value. If you want to use the result of an evaluated expression later in your program, you can save it inside a variable. You’ll store values in variables with an assignment statement. An assignment statement consists of a variable name, an equal sign, and the value to be stored. In Python, every single thing is stored as an object. A Python variable is actually a reference to an object!
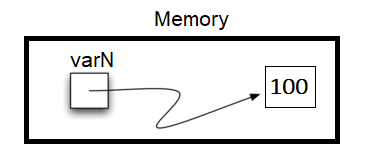
A variable is initialized (or created) the first time a value is stored in it. After that, you can use it in statements with other variables and values. When a variable is assigned a new value, the old value is forgotten. This is called overwriting the variable.
spam = 'Hello' # 'Hello' is a string object
print(spam) # spam is a variable, it is just a reference or tag
spam = 'Goodbye' # 'Goodbye' is another string object
print(spam)
Hello
Goodbye
It’s much better to think of variables as labels that you can assign to values. You can also say that a variable references a certain value.
The naming of variables is largely up to the user in Python. Python’s simple rules are that variable names must begin with an alphabet letter or the underscore character, and may consist of an arbitrary number of letters, digits, and the underscore character (A-z, 0-9, and _ ). While a variable named m
may be sufficient for a two line example script, experienced programmers strive to give meaningful, descriptive variable names in their scripts.
Valid variable names | Invalid variable names |
---|---|
current_balance | current-balance (hyphens are not allowed) |
currentBalance | current balance (spaces are not allowed) |
account4 | 4account (can’t begin with a number) |
_42 | 42 (can’t begin with a number) |
TOTAL_SUM | TOTAL_$UM (special characters like $ are not allowed) |
hello | ‘hello’ (special characters like ’ are not allowed) |
Python variable names are case-sensitive, meaning that capitalization matters. A variable named size
is treated as distinct from variables named Size
or SIZE
. A small number of keywords, names that are reserved for special meaning in Python, cannot be used as variable names. You can view this list by accessing the built-in Python help system.
Here is a list of the Python keywords. Enter any keyword to get more help.
False class from or
None continue global pass
True def if raise
and del import return
as elif in try
assert else is while
async except lambda with
await finally nonlocal yield
break for not
Variables can be used to store all of the types of data values that Python is able to represent.
my_string = 'characters'
my_Boolean = True # True/False
my_integer = 5
my_floating_point = 26.2
my_complex = 2+1j # Note that 1 can not be omitted
# You can condense the above statements into one line separated by ;
my_string = 'characters'; my_Boolean = True; my_integer = 5; my_floating_point = 26.2; my_complex = 2+1j
### Multiple Assignment!
# You can also assign values to more than one variable using just a single line of code!
my_string, my_Boolean, my_integer, my_floating_point, my_complex = 'characters', True, 5, 26.2, 2+1j
print(10)
print(3.14)
print(2e10) # scientific notation
print(12_000) # you can group digits using underscores to make large numbers more readable
print(3+2j)
10
3.14
20000000000.0
12000
(3+2j)
Note that when you’re writing long numbers, you can group digits using underscores to make large numbers more readable. In addition, print()
can be used to print any numerical number including those in scientific notation.
1.2.1 Data types
In Python variables and constants have a type. We can ask Python what type something is by using the type()
function
(str, bool, int, float, complex)
You can convert object of one type to another using cast by str()
, float()
, int()
, etc.
Python ord()
and chr()
are built-in functions. They are used to convert a character to an int and vice versa. Python ord()
and chr()
functions are exactly opposite of each other.
Python ord()
function takes string argument of a single Unicode character and return its integer Unicode code point value. Let’s look at some examples of using ord()
function.
Python chr()
function takes integer argument and return the string representing a character at that code point.
1.2.1.1 Conversion
There are two types of type conversion in Python.
- Implicit Conversion - automatic type conversion. Python always converts smaller data types to larger data types to avoid the loss of data.
- Explicit Conversion - manual type conversion
In Python, complex > float > int > bool
1.2.2 Debugging
Programming languages are not very forgiving for beginners, and a great deal of time learning to write software can be spent trying to find bugs, or errors in the code. Locating such bugs and correcting them is thus known as debugging. There are three major classes of bug that we create in software: syntax errors (mistakes in the symbols that have been typed), semantic errors (mistakes in the meaning of the program), and runtime errors (mistakes that occur when the program is executed.)
Syntax errors are the most common for novices, and include simple errors such as forgetting one of the quote marks at the beginning or ending of a text string, failing to close open parentheses, or misspelling the function name print()
. The Python interpreter will generally try to point these errors out when it encounters them, displaying an offending line number and a description of the problem. With some practice, a beginner can quickly learn to recognize and interpret common error cases. As examples:
This expression is missing a value between the addition operator and the closing parenthesis.
In this case it found a name error and reports that the variable being printed has not been defined. Python can’t identify the variable name provided.
Like calling someone by the wrong name, misspelling the name of a known function or variable can result in confusion and embarrassment.
Semantic errors are flaws in the algorithm, or flaws in the way the algorithm is expressed in a language. Examples might include using the wrong variable name in a calculation, or getting the order of arithmetic operations wrong in a complex expression. Python follows the standard rules for operator precedence, so in an expression like total_pay = 40 + extra_hours * pay_rate
, the multiplication will be performed before the addition, incorrectly calculating the total pay. (Unless your pay rate happens to be $1/hour.) Use parenthesis to properly specify the order of operations in complex expressions, thereby avoiding both semantic errors and code that may be harder to understand (e.g., total_pay = (40 + extra_hours) * pay_rate
).
Finally, runtime errors at this level might include unintentionally dividing by zero or using a variable before you have defined it. Python reads statements from top to bottom, and it must see an assignment statement to a variable before that variable is used in an expression.
1.3 The first program
While the interactive shell is good for running Python instructions one at a time, sometimes you have to use a script, to write entire Python programs. In this case, you’ll type the instructions into the file editor.
%%writefile hello.py
"""
This program says hello and asks for your name.
It also ask the age of you.
"""
print('Hello, world!')
myName = input('What is your name? ') # ask for their name
print('It is good to meet you, ' + myName)
print('The length of your name is:\n' + str(len(myName)))
myAge = input('What is your age? ') # ask for their age
print('You will be ' + str(int(myAge) + 1) + ' in a year.')
Writing hello.py
Once you’ve entered your source code, the ipython magic %%writefile
will save it so that you won’t have to retype it each time you start. You can then use another magic %run
to execute the python script.
Hello, world!
It is good to meet you, phonchi
The length of your name is:
7
You will be 33 in a year.
1.3.1 Dissecting Your Program
With your new program open in the file editor, let’s take a quick tour of the Python instructions it uses by looking at what each line of code does.
The first line is the comment that tells others your intention of this program or the authorship of the program. Here it uses the multiline comment by using the triple quotes. The line
print('Hello, world!')
means “Print out the text in the string ‘Hello, world!’.” When Python executes this line, you say that Python is calling theprint()
function and the string value is being passed to the function. A value that is passed to a function call is an argument. When you write a function name, the opening and closing parentheses at the end identify it as the name of a function.The
input()
function waits for the user to type some text on the keyboard and press ENTER. ThemyName = input()
function call evaluates to a string equal to the user’s text, and the line of code assigns themyName
variable to this string value.The following call to
print('It is good to meet you, ' + myName)
is an expression.'It is good to meet you, '
andmyName
between the parentheses are concatenated together via the operand+
and printed out.You can pass the
len()
function a string value (or a variable containing a string), and the function evaluates to the integer value of the number of characters in that string. If you want to concatenate an integer such as 29 with a string to pass toprint()
, you’ll need to get the value ‘29’, which is the string form of 29. Thestr()
function can be passed an integer value and will evaluate to a string value version of the integer. Besides, there is a\n
in the statementprint('The length of your name is:\n' + str(len(myName)))
. The string starts with the backslash has a special escape meaning and\n
means adding a newline.The
int()
function is also helpful if you have a number as a string value that you want to use in some mathematics. For example, theinput()
function always returns a string, even if the user enters a number. ThemyAge
variable contains the value returned frominput()
. You can use theint(myAge)
code to return an integer value of the string inmyAge
. This integer value is then added to 1 in the expressionint(myAge) + 1
. The result of this addition is passed to thestr()
function:str(int(myAge) + 1)
. The string value returned is then concatenated with the strings'You will be '
and' in a year.'
to evaluate one large string value. This large string is finally passed toprint()
to be displayed on the screen.
1.3.2 Exercise 2: Write a script that inputs a five-digit integer from the user. Separate the number into its individual digits. Print them separated by three spaces each. For example, if the user types in the number 42339, the script should print 4 \(~~\) 2 \(~~\) 3 \(~~\) 3 \(~~\) 9
Hint: Use floor division (//
) and remainder (%
) to isolate the digits.
# Your answer here
# x=42339
# Get the user's input from their keyboard and convert it to integer:
x = _____('Enter a 5 digit integer')
# Get the last digit by remainder
digits4 =
# Perform floor division and get the remainig digits
x =
#....
# Print out the results
____(digits0,' ',digits1,' ',digits2,' ',digits3,' ',digits4)
4 2 3 3 9
1.4 The zen of Python
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
You can compute expressions with a calculator or enter string concatenations with a word processor. You can even do string replication easily by copying and pasting text. But once you know how to handle the programing elements, you will be able to instruct Python to operate on large amounts of data automatically for you to replace these tasks.
Don’t try to write perfect code; write code that works, and then decide whether to improve your code for that project or move on to something new. But as you continue to the next chapters and start digging into more involved topics, try to keep this philosophy of simplicity and clarity in mind.
You can also refer PEP8 which is a reference document that provides guidelines and best practices on how to write Python code.