(-1.0,)
Appendix A — Increase productivity and learning with Github Copilot in Python
|
|
A.1 Get Started With GitHub Copilot in Python
GitHub Copilot offers a virtual AI-powered assistant integrated into your code editor, creating significant buzz upon its public release. This tool provides exceptional support for languages such as Python
.
GitHub Copilot is the first commercial product based on the OpenAI Codex system, capable of converting natural language into code across more than a dozen programming languages. OpenAI Codex comes from the GPT-3 language learning model. It was taught using both regular text and a vast number of code examples found on GitHub, which has hundreds of millions of public code projects.
A.1.1 Trial or get your free access to GitHub Copilot
You can enjoy a thirty-day trial period without paying anything, but only after providing your billing information (Be sure to cancel the unpaid subscription plan before it expires to avoid unwanted charges!).
Students can get a free GitHub Copilot subscription. GitHub will verify your status once a year based on proof of academic enrollment, such as a picture of your school ID or an email address in the .edu
domain. For detailed instructions on setting up, follow the steps in our notes.
A.1.2 Install a Visual Studio Code Extension
Because Microsoft owns GitHub, it’s no surprise that their Visual Studio Code editor was the first tool to receive GitHub Copilot support. There are a few ways to install extensions in Visual Studio Code, but the quickest is that you can find the Extensions icon in the Activity Bar located on the left-hand side of the window and try searching for the “GitHub Copilot” and “Github Copilot Labs” extension on the Visual Studio Marketplace.
After the installation is complete, Visual Studio Code will ask you to sign in to GitHub to give it access to your GitHub profile, which your new extension requires. Visual Studio Code needs to know who you are to verify your GitHub Copilot student status.
However, granting access to your GitHub profile will allow the editor to read your private repositories. If you change your mind, then you can revoke this authorization at any time by going to your GitHub profile settings and finding GitHub for VS Code in the Authorized OAuth Apps.
A.1.3 Keyboard shortcut
To make working with GitHub Copilot in Visual Studio Code even more productive, here are the most common keyboard shortcuts worth remembering:
Action | Windows / Linux | macOS |
---|---|---|
Trigger inline suggestions | Alt+\ | Option+\ |
See the next suggestion | Alt+] | Option+] |
See the previous suggestion | Alt+[ | Option+[ |
Accept a suggestion | Tab | Tab |
Dismiss an inline suggestion | Esc | Esc |
Show all suggestions in a new tab | Ctrl+Enter | Ctrl+Enter |
You can change the keyboard shortcut by going to File → Preferences → Keyboard Shortcuts or Code → Preferences → Keyboard Shortcuts on macOS.
Sometimes GitHub Copilot suggestions may get in your way. If that’s the case, then you can disable them globally or for a particular programming language by clicking on the extension’s icon in the bottom right corner of the editor’s window:
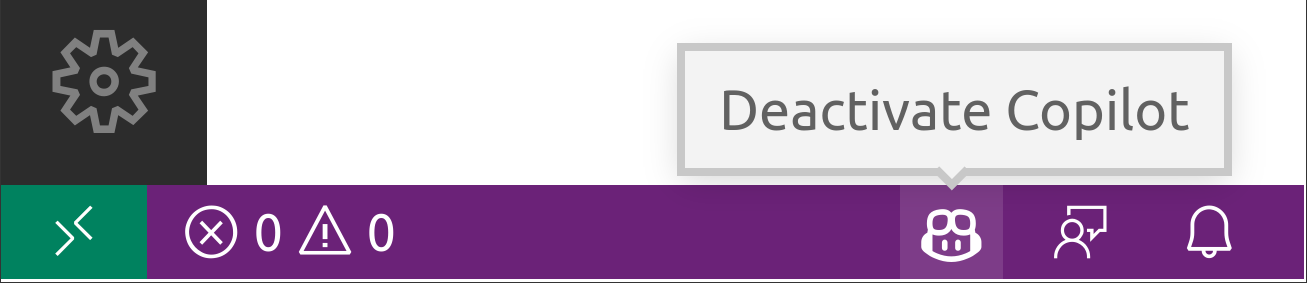
A.1.4 Hand Over the Reins to GitHub Copilot
Now it’s time to make sure GitHub Copilot is working as expected in either Visual Studio Code. To check if GitHub Copilot is working as expected start writing a sample function signature, such as def hello
:
As soon as you type the colon (:
) at the end of the first line to introduce a new code block, GitHub Copilot fills in the suggested function body for you. Until you either accept it by hitting Tab
or reject it with Esc
, it’ll show up in gray font. The suggested code calls the print()
function to display the Hello World text on the screen in this case. While that wasn’t spectacular, it confirms that GitHub Copilot is working correctly! Now let’s try another function signature such as def add(a, b)
:
Sure enough, GitHub Copilot gives a very sensible suggestion, which returns the sum of a
and b
. Notice the difference between returning a value from the function and printing the result on the screen. Your clever virtual assistant can infer the intent from the function’s name and arguments!
A.2 Synthesize Python Code From Natural Language
Because GitHub Copilot was trained in natural language and curated samples of different programming languages, it’s perfectly possible to explain an abstract problem using plain English or another natural language and expect it to generate the corresponding code in the desired programming language.
The underlying machine learning model is also capable of doing the opposite — explaining a piece of code in a natural language or even translating one programming language into another, as we will see later on!
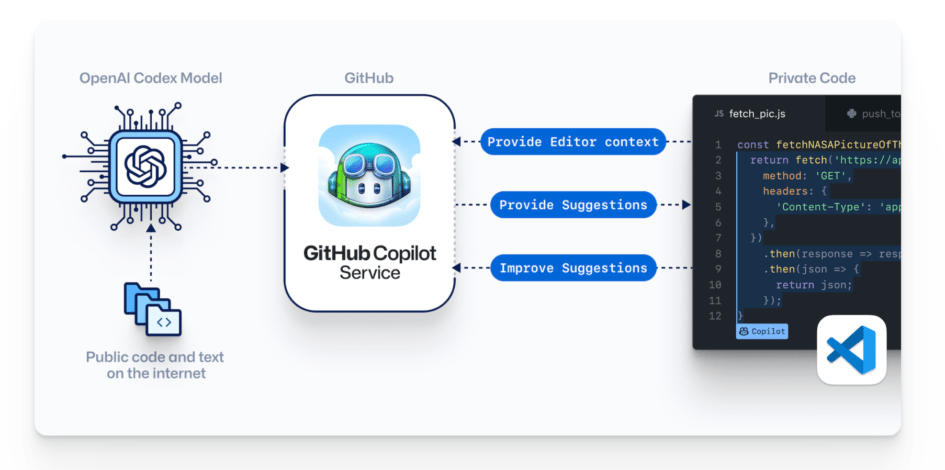
A.2.1 Use a Python Comment to Describe the Problem
Comments can sometimes help explain why a certain piece of code looks the way it does. You typically write comments for your future self or your teammates working on the same codebase. When you add GitHub Copilot into the mix, it becomes another target audience who can read your code comments. Consider the following single-line comment in Python
, which describes the classic Hello, World! Program:
After typing that comment into your code editor, you’ll notice that GitHub Copilot doesn’t automatically pick it up. When you choose to communicate with it through comments, you must sometimes
Open the GitHub Copilot side panel or tab to see the suggestions (
CTRL+ENTER
)Alternatively, you may start typing a bit of code to have it auto-completed.
Notice that GitHub Copilot understands that you wish to treat the quoted fragment "Hello, world!"
of your comment as literal text rather than an instruction!
Apparently, that was too easy for GitHub Copilot. How about raising the bar by requesting a more specific output? For example, you may want to print Hello, World! backward in Chinese.
Now, that’s impressive! GitHub Copilot nails it by generating not only correct but also Pythonic code that experienced Pythonistas would write themselves. The suggestions will get even more interesting when you include more comments.
A.2.2 Add More Comments to Increase the Problem Complexity
Using a single-line comment to describe a problem is fine, but you can only pack so much content in it. Fortunately, it’s possible to combine multiple consecutive comments into a logical and cohesive story that GitHub Copilot will treat as a whole. You can use multiline comments to achieve this:
"""
Write a program that does the following:
1. Create a dictionary called `grades` that maps subject names to lists of grades for each student. Assume that each student has the same number of grades for each subject.
2. Create a dictionary called `subject_averages` that maps subject names to their average grades across all students.
3. Create a dictionary called `student_totals` that maps student names to their total grades across all subjects.
4. Create a variable called `top_student` that contains the name of the student with the highest total grade.
5. Print out the top-performing student's name and total grade, as well as the average grade for each subject.
"""
grades = {
'Math': [[90, 85, 92, 87, 94], [95, 88, 91, 89, 92], [87, 83, 85, 90, 89]],
'Science': [[82, 88, 89, 91, 85], [90, 86, 88, 87, 92], [85, 83, 87, 84, 89]],
'English': [[88, 85, 92, 86, 90], [91, 87, 89, 92, 85], [83, 86, 88, 87, 90]],
}
Isn’t that amazing? You gave GitHub Copilot a natural language description of a task, and it got the right solution for you.
Keep in mind that the suggestions you’ll might get different results from those presented here. Sometimes it takes trial and error before getting the desired result, so try tweaking your comments a little bit if you’re not getting satisfactory results immediately.
You can edit the code generated by GitHub Copilot just like your own code. Sometimes, you may not like its formatting, the naming conventions it applied, or a specific fragment of the logic you’d instead rewrite. On other occasions, seeing a suggestion may inspire you to come up with a more clever alternative.
A.2.3 Receive Even More Intelligent Code Completion Suggestions
You can think of GitHub Copilot as an intelligent code completion mechanism on steroids that understands the context of your project at a deep level, providing the most appropriate suggestions for you. When you work with GitHub Copilot long enough, it may sometimes give you the creepy feeling that it can read your mind. Say that you want to find the roots of the second-degree polynomial, also known as the quadratic function, with three coefficients: a
, b
, and c
:
A.2.3.1 Provide Context to Get Better Suggestions
The fundamental theorem of algebra states that a degree 𝑛 polynomial with complex coefficients has exactly n
complex roots. In other words, a quadratic function, which is a second-degree polynomial, always has exactly two complex roots even when there are none in the real domain. How can you request that GitHub Copilot change the implementation so that you’ll get the complex roots instead of the real ones? You need to add constraints to the problem by giving GitHub Copilot some context to draw from. For example, you may import a module that you wish to be used or write a Python
docstring that describes the expected result in natural language:
A.2.4 Always Have the API Documentation at Your Fingertips
Say you wanted to write a small Python
function to process the word file. In the traditional approach, you’d start by making a web search for word API. Then, you’d probably get overwhelmed by their guides, quick starts, and reference documentation. Fortunately, you have GitHub Copilot, which has been trained to use well-known APIs, so you can give it a minimal hint about which API to call:
# Install library that process word file for python
# Download the word file from 'https://asc.nsysu.edu.tw/var/file/10/1010/img/3362/344961432.docx' using Python
# Getting the Full Text from a demo.docx File
Exploring new libraries in Python with GitHub Copilot is an enjoyable experience. Perhaps you’re revisiting a framework for which your knowledge has become a little rusty, and you’re struggling to remember where to begin. But even when you’re familiar with a given tool, GitHub Copilot can sometimes surprise you by suggesting more efficient solutions or parts of its API you had no idea about.
The name Copilot was a clever choice by GitHub to describe this product. It avoids misleading people into thinking it could take control and replace you as the programmer. You must remember that the code produced by GitHub Copilot isn’t always ideal. It can sometimes be suboptimal or insecure, and it might follow bad programming practices.
It’s less of a problem when the code contains syntactical errors or if it’s completely nonsensical because that’s easy to spot. However, the code that looks plausible at first glance can still be incorrect and contain logical errors. Therefore, you should never trust GitHub Copilot without carefully reviewing and testing the code it generates!
It may make us lazy and discourage us from thinking independently. Like with every powerful tool, you can use GitHub Copilot for right or wrong purposes.
GitHub Copilot is a revolutionary programming aid that can increase your speed and productivity as a software engineer. It saves you time by generating boilerplate code and sparing you from diving into documentation. Because it understands the context of your project, the instant suggestions you get are tailor-made and usually work the way you intended.
A.2.5 Exercise 1: Install the pdfplumber
, requests
and pandas
libray. Then download the file from the internet “https://rpb28.nsysu.edu.tw/static/file/183/1183/img/1373/625717409.pdf” using Python
. Finally, extract the table from the pdf file and organize it into a table by excluding the first column and newline.
A.2.6 Prompt-engineer
Try
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29]
Try
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29]
A.3 Copilot Labs
Try the following program from the midterm:
def isLeapYear(year):
# Your code here
# Years divisible by 400 are leap years:
if ____:
return True
# Otherwise, years divisible by 100 are not leap years:
elif ____:
return False
# Otherwise, years divisible by 4 are leap years:
elif ____:
return True
# Otherwise, every other year is not a leap year:
else:
return False
# Use a while loop and isLeapYear() to determine the upcomming year
def upcoming_leap_year(current_year):
# Your code here
____
current_year += 1
assert upcoming_leap_year(2023) == 2024
assert upcoming_leap_year(1999) == 2000
assert upcoming_leap_year(2099) == 2104